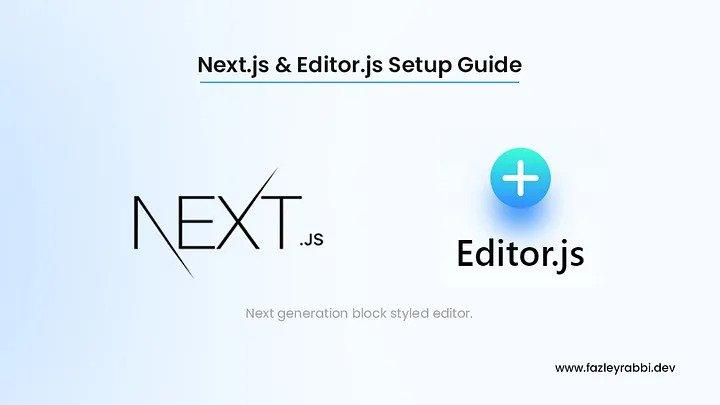
In this article, I’m gonna show you how you can set up Editor.js In your Next.js project. I’ve faced some problems adding Editor.js with Next.js. With the help of Stack Overflow & GitHub, I’ve figured out how to implement it. This is gonna be my complete guide of Editor.js with Next.js.
For those who don’t know about Editor.js, It’s block styled editor. You can read about it here.
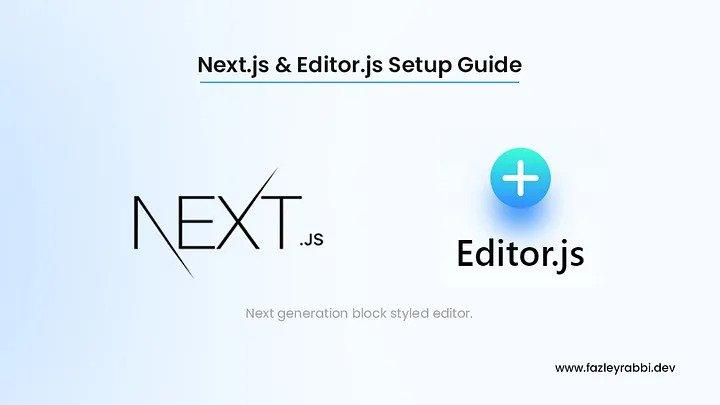